Goal:
Learn javascript. Improve my javascript fundamentals and in general improve my knowledge of vanilla js.
Tech Stack:
- PURE JS BABY
Recap:
I had a mock interview conducted by a good friend who works as a senior dev and tech consultant who often conducts interviews for his company. It was my first interview experience period so I had a lot to learn and was glad that he was gentle with me throughout the process. He told me I did very well but really I think he's just being nice, I did alright but not spectacularly. There's a lot of fundamentals and knowledge base that I lack and the lack of confidence in those areas shows as well. I took a break from react and making websites this week, sort of at least, to focus on the nitty gritty leetcode, codewars, quizlet life.
Here's how it went:
Q1:
Timmy & Sarah think they are in love, but around where they live, they will only know once they pick a flower each. If one of the flowers has an even number of petals and the other has an odd number of petals it means they are in love.
Write a function that will take the number of petals of each flower and return true if they are in love and false if they aren't.
My Solution:
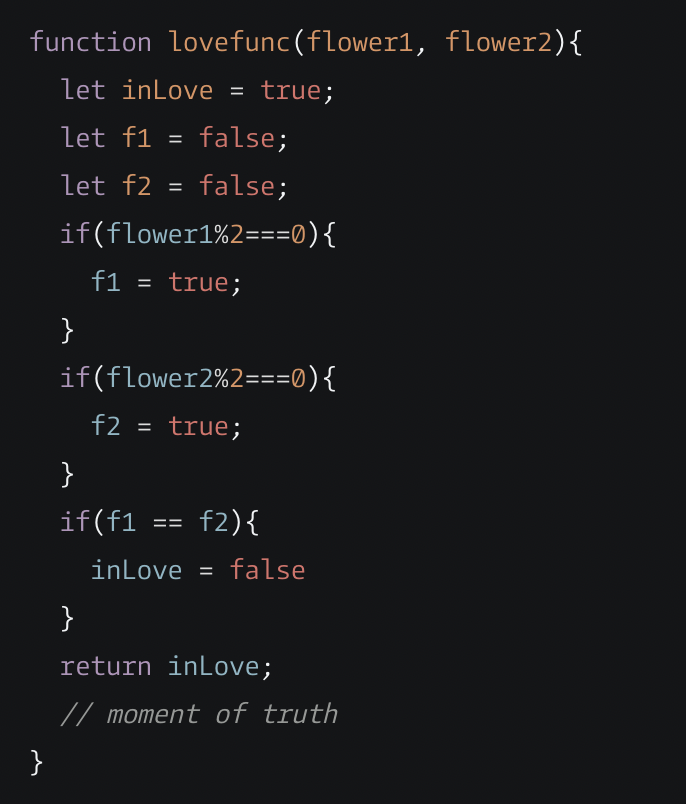
I knew that a lot of these questions in the beginning wouldn't be too challenging to do, it was really about pushing myself to find smarter, faster, better ways of doing things. The thing I like about codewars is the ability to look at other people's solutions. I can very quickly see what others did that was better and what I need to learn, without being able to see more competent dev's solutions there would be lots of strategies and functions that I didn't even know I didn't know.
A better refactored solution that I saw:
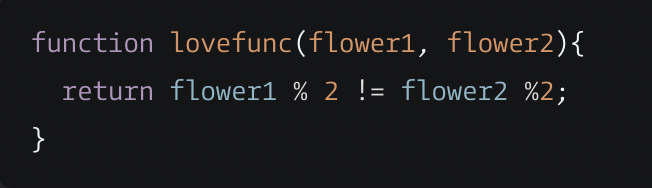
What I learned:
I'm an idiot who over thinks things. On a more serious note, I realized that I don't need to create a separate boolean to return, there are plenty of evaluations that will naturally return true or false and I can shorten my code by returning the evaluations directly.
Q2:
Given an array of integers.
Return an array, where the first element is the count of positives numbers and the second element is sum of negative numbers. 0 is neither positive nor negative.
If the input is an empty array or is null, return an empty array.
My Solution:

Refactored Solution 1:

Thoughts:
Holy crap is this what senior devs think like? Or just competent devs? I have never used filter, or reduce before and I rarely use ternary operators though I know how that works at least. Learned nothing because was too advanced really, in my current state would never think like that.
Refactored Solution 2:
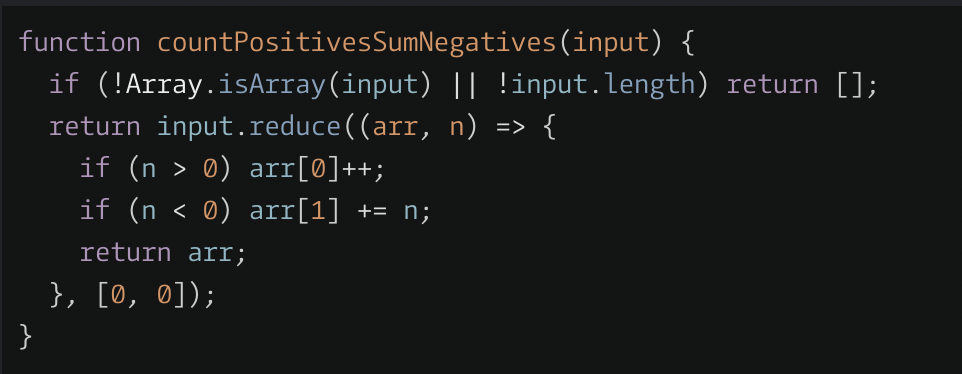
Thoughts:
Look up reduce in MDN, same logic that I used but just more efficient and less lines of code. Also need to ask someone else on best practices, I thought it was generally less good to have multiple return statements even if more efficient?
Q3:
Write function bmi that calculates body mass index (bmi = weight / height2).
if bmi <= 18.5 return "Underweight"
if bmi <= 25.0 return "Normal"
if bmi <= 30.0 return "Overweight"
if bmi > 30 return "Obese"
My Solution:
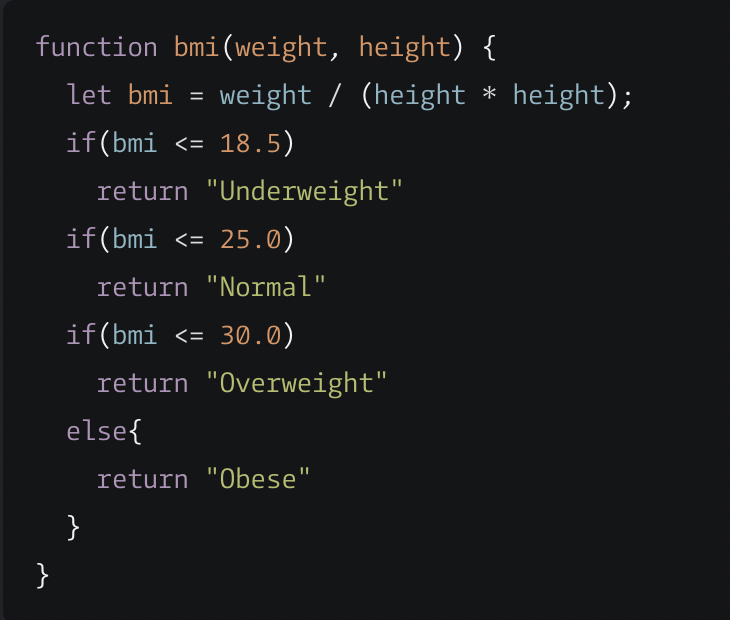
Refactored Solution:

Thoughts:
Destructuring and nested ternary operators cool beans, faster quicker code. Note to self, get better with ternary operators!
Q4:
Build a function that returns an array of integers from n to 1 where n>0.
Example : n=5 --> [5,4,3,2,1]
My Solution:

Refactored:

Thoughts:
This is a really cool solution, I've only used Array.from once and I never really got the hang of it, it really feels like a good solution but requires a really good knowledge of the different components and being able to make use of every moving element. This is sort of the level of understanding that I'd like to get to.
Q5:
Implement a function which convert the given boolean value into its string representation.
Note: Only valid inputs will be given.
My Solution:

Thoughts:
There were a surprising number of other solutions all of which seemed significantly worse? I can't help but wonder if it's just an es6 implementation or whether some of these answers were from a long long time ago.
Q6:
Clock shows h hours, m minutes and s seconds after midnight.
Your task is to write a function which returns the time since midnight in milliseconds.
My Solution:

Thoughts:
Again, pretty simple problem, no answers that really wowed me or impressed me. At most some solutions were maybe slightly more efficient to write but simply way worse to read and overly complex for truly a very easy problem.
Q7:
Very simple, given an integer or a floating-point number, find its opposite.
My Solution:
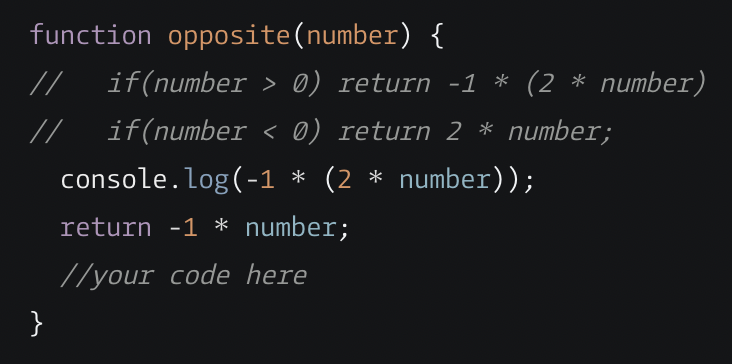
This isn't a hard question...which makes it hard because I stressed a bit too much about finding a clever solution. Also I got a bit mixed up with negatives and double negatives. Again, this should be a 1 min problem but took me a bit longer than that truthfully.
Refactored:
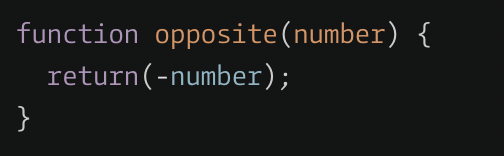
Thoughts:
:( I was so sure there was a clever efficient solution that I completely missed the clever efficient solution and made things so much more complicated for myself. I'm also literally like the only person online who didn't immediately find this solution by the way ....
Q8:
Sum all the numbers of a given array ( cq. list ), except the highest and the lowest element ( by value, not by index! ).
The highest or lowest element respectively is a single element at each edge, even if there are more than one with the same value.
Mind the input validation.
My Solution:
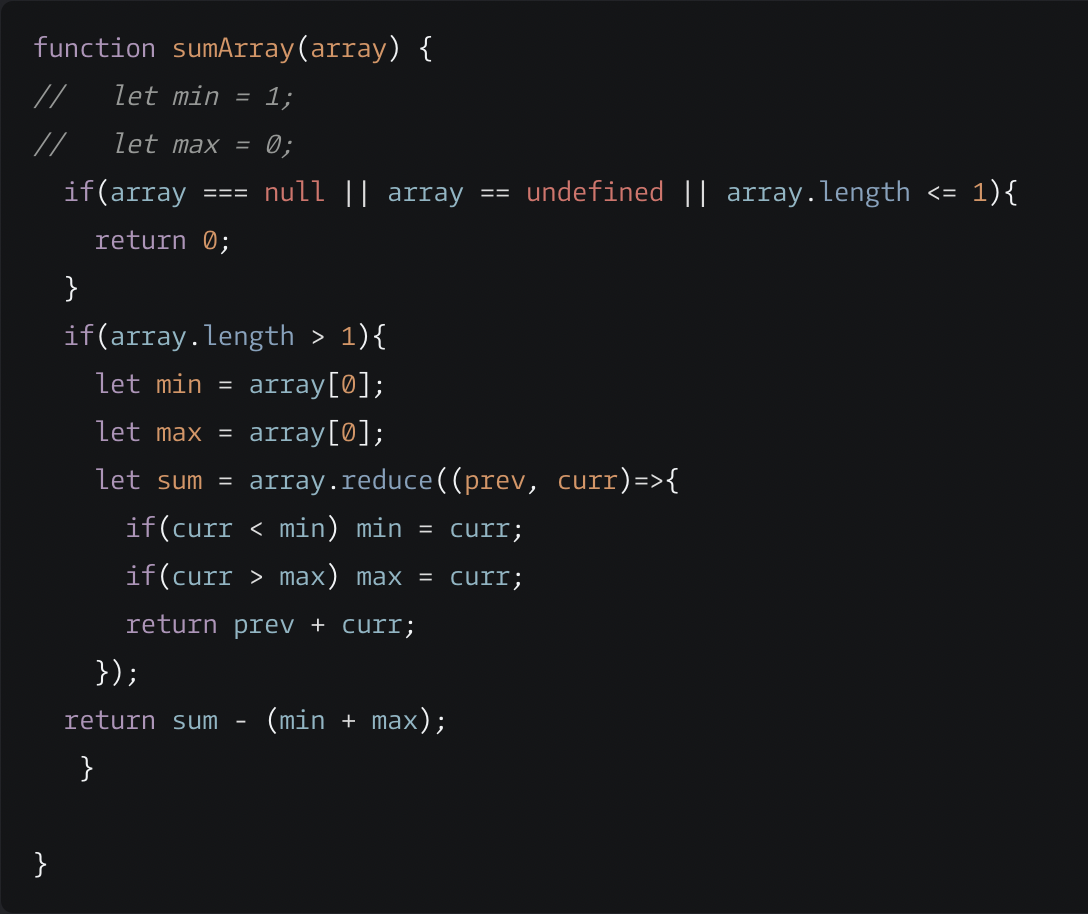
Trying to push myself to use reduce more and familiarize myself with more of what's available to me. This problem is a mix of classics, find the sum of an array and find the min and max of an array w/ a sprinkle of input validation.
Refactored 1:

Thoughts:
Uh wow truly so much going on. Was happy that I even used reduce.
Refactored 2:

Thoughts:
I like this one a lot more because it's the same logic and approach I went with just with more efficient syntax and methodology. Now I know I can just Math.min or Max an array from spread operator.
Q9:
Your task is to find the first element of an array that is not consecutive.
By not consecutive we mean not exactly 1 larger than the previous element of the array.
E.g. If we have an array [1,2,3,4,6,7,8] then 1 then 2 then 3 then 4 are all consecutive but 6 is not, so that's the first non-consecutive number.
If the whole array is consecutive then return null2.
The array will always have at least 2 elements1 and all elements will be numbers. The numbers will also all be unique and in ascending order. The numbers could be positive or negative and the first non-consecutive could be either too!
My Solution:

Took a little more bug fixing than I would have liked, couldn't find a clever way to make sure the conditional ran once so jokingly created a 'bandaid' boolean to control when it triggers.
Refactored:
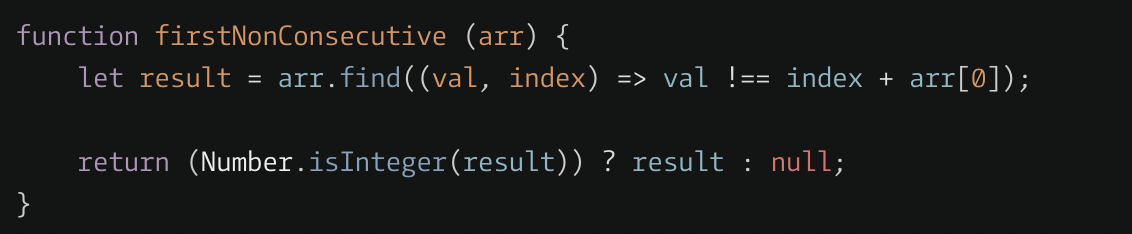
Thoughts:
I seriously need to learn more about array methods. And also push myself to use ternary!!!!
Q10:
Complete the solution so that it reverses the string passed into it.
My solution:

I felt pretty good about this, going into it I knew for sure how I could complete this with some forloops and multiple new strings etc but because this is a classic question I wanted to find a way that might show off what I know to an interviewer/be more efficient.
Refactored:

Thoughts:
IM THE ONLY PERSON WHO DIDN"T KNOW THIS OR HTINKOF THIS >_< AHHHHHHHHHHHHHHH I TRIED TO GET FANCY AND I WAS PUNISHED
Q11:
Check to see if a string has the same amount of 'x's and 'o's. The method must return a boolean and be case insensitive. The string can contain any char.
My Solution:

Refactored:

Thoughts:
On one hand I'm glad I have the logic to complete these tasks...but it's really apparent that I don't know my string and array fundamentals. There are just easier ways and existing methods of doing these things and I'm not taking advantage of them because I'm not aware of them.
NEED TO FIX ASAP